K-Type Thermocouple MCP9600 with Arduino
Thermocouples are one of the most interesting electronic components. If you are looking for a high accuracy, wide range, high temperature, low temperature, low cost industrial grade thermocouple device then this article is for you. In this article we will be using K-type thermocouple with a MCP9600. This K-type thermocouple uses the MCP9600 and may be used in high temperature application like temperature measurement of an oven, heating plates, boiler temperature measurement, grill temperature measurement and much more.
To Interface K-type thermocouple MCP9600 with Arduino you will need following hardware, all these parts can be found on ncd.io
- MCP9600
- Arduino I2C Shield
- Arduino
K type thermocouple MCP9600 Board Overview
The thermocouple board comes with one I2C cable, one k-type thermocouple and the MCP9600 mini module. The board is small in size and comes with mounting holes, which makes installation real easy. The length of thermocouple wire is around 44 inches (112 cm). The long wire comes in real handy during installation. You could put the board far away from the high temperature and use the thermocouple to read the temperature. You can use this thermocouple to measure the temperature of a liquid, for example you can use it with a relay controller and turn on off a heater which heats up the water, so in this case you can use this thermocouple to measure the liquid temperature and heat it up at a fixed temperature.
This K-type thermocouple MCP9600 talks to master devices using I2C communication and it usages analog addressing, so on the board there is a potentiometer which you can use to change the I2C address. This MCP9600 is one of the best thermocouple chip available in the market, you can connect up to 8 of these boards on one I2C bus. If you want to connect more then 8, then you will need to use an I2C multiplexer.
Legends say this thermocouple is so good that you can use it to measure your ex’s heart temperature, which is indeed very low(cold), as well as your current partner’s temperature which we hope is real High (Hot hot).
K type thermocouple MCP9600 Arduino code
The MCP9600 is loaded with a lot of features which makes it a great chip but at the same time that makes it little difficult to use. Once you go through the datasheet, you will be able to understand the MCP9600 functionality and the arduino code will make a lot more sense.
You can find the MCP9600 datasheet over here
You can find the k-type thermocouple MCP9600 Arduino code over here
#include<Wire.h> #define Addr 0x64 long data1; long data2; long temp; byte stat; void setup() { // Initialise I2C communication Wire.begin(); // Initialise Serial Communication, set baud rate = 9600 Serial.begin(9600); temrmo_set(); device_set(); } ///// this function will read the hot and cold junction temp int read_temp() { Wire.beginTransmission(Addr); Wire.write(0x00); Wire.endTransmission(); Wire.requestFrom(Addr, 2); if (Wire.available() == 2) { data1 = Wire.read(); data2 = Wire.read(); // Serial.println(data1); // Serial.println(data2); if((data1 & 0x80) == 0x80) { data1 = data1 & 0x7F; temp = 1024 - ( data1 * 16 + data2/16); Serial.print("Temperature :"); Serial.print(temp); Serial.println("n"); } else data1 = data1 *16; data2 = data2 * 0.0625; // Serial.println(data1); // Serial.println(data2); temp = data1 + data2; // temp = ( data1 * 16 + data2/16); Serial.print("Temperature :"); Serial.print(temp); Serial.println("n"); } } /// this function can be used to see if the temp conversion is complete or not int temp_stat() { Wire.beginTransmission(Addr); Wire.write(0x04); Wire.endTransmission(); delay(50); Wire.requestFrom(Addr, 1); if (Wire.available() == 1) { stat = Wire.read(); } // Serial.print(stat); return stat; } /// this functon can be used to clear the temp conversion flag int temp_stat_clr() { Wire.beginTransmission(Addr); Wire.write(0x04); Wire.write(0x0F); Wire.endTransmission(); } ////// this function can be used to set the thermocuple configuration int temrmo_set() { Wire.beginTransmission(Addr); Wire.write(0x05); Wire.write(0x00); Wire.endTransmission(); } ////////////// this function can be used to set the device config int device_set() { Wire.beginTransmission(Addr); Wire.write(0x06); Wire.write(0x00); Wire.endTransmission(); } void loop() { temp_stat(); byte thermo_ready = (stat && 0x40); if(thermo_ready) { read_temp(); // Serial.print("hello"); } temp_stat_clr(); delay(1000); }
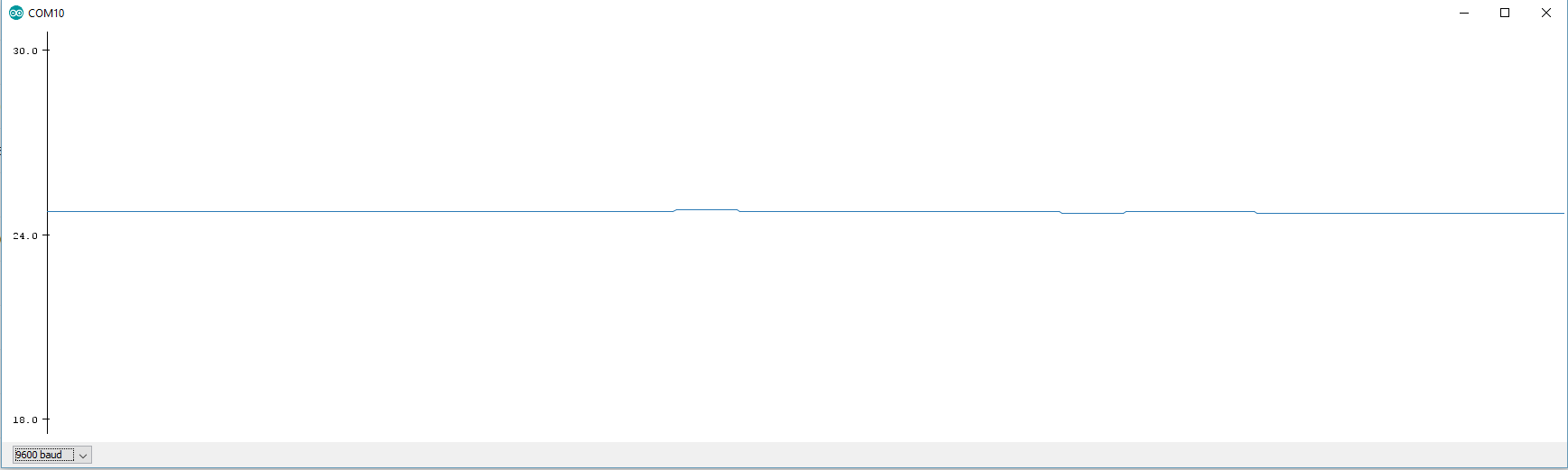
In this code we have 5 main function
- Read k-type thermocouple mcp9600 temperature
this function is used to read the temperature
///// this function will read the hot and cold junction temp int read_temp() { Wire.beginTransmission(Addr); Wire.write(0x00); Wire.endTransmission(); Wire.requestFrom(Addr, 2); if (Wire.available() == 2) { data1 = Wire.read(); data2 = Wire.read(); // Serial.println(data1); // Serial.println(data2); if((data1 & 0x80) == 0x80) { data1 = data1 & 0x7F; temp = 1024 - ( data1 * 16 + data2/16); Serial.print("Temperature :"); Serial.print(temp); Serial.println("n"); } else data1 = data1 *16; data2 = data2 * 0.0625; // Serial.println(data1); // Serial.println(data2); temp = data1 + data2; // temp = ( data1 * 16 + data2/16); Serial.print("Temperature :"); Serial.print(temp); Serial.println("n"); } }
in this function we read the temperature, the temperature register location is 0x00. This function can be used to read hot junction temperature as well as cold junction temperature. Hot junction temperature is the temperature which is above 0’C and cold junction temperature is the temperature which is below 0’C. This function will automatically detect he hot and cold junction conditions and will calculate the temperature based on those settings.
2. Read thermocouple status
This function is one of the most important function, this function is used to check if the MCP9600 is done with taking the temperature samples and conversion, if this function is not implemented you will see weird results coming out from the MCP9600.
/// this function can be used to see if the temp conversion is complete or not int temp_stat() { Wire.beginTransmission(Addr); Wire.write(0x04); Wire.endTransmission(); delay(50); Wire.requestFrom(Addr, 1); if (Wire.available() == 1) { stat = Wire.read(); } // Serial.print(stat); return stat; }
The thermocouple status register location is 0x04, so to know the thermocouple status you will need check this register value, once you have the register value you will need to check 6th bit, if this bit is high it means the MCP 9600 is done with the temperature conversions and its ready to read.
3. Thermocouple sensor configuration
////// this function can be used to set the thermocuple configuration int temrmo_set() { Wire.beginTransmission(Addr); Wire.write(0x05); Wire.write(0x00); Wire.endTransmission(); }
This function can be used to set the sensor type, this chip supports a few kinds of thermocouple so you will need set what kind of thermocouple you are connecting with the MCP9600, in this case we care connecting K type, so we will set the 0x05 register value to 0x00. this register can also be used to set the filters, in this code we are not using any filters.
4. MCP9600 Device configuration
//header to access SPIFFS #include "FS.h" //include this in setup() SPIFFS.begin(); //get the webform file from SPIFFS File file =SPIFFS.open("/webform.html", "r"); server.streamFile(file,"text/html"); //don't forget to close the file file.close();
This function will set the device settings like hot cold junction, resolution, number of samples and mode of operation. these settings can help you increasing the data frequency and the temperature results accuracy as well.
Reading extremely high temperature using K type thermocouple MCP9600 and Arduino
There are a lot of thermocouples which comes with 4-20mA current loop output and if you are looking for such thermocouple interface please check out this article
Readings 4-20mA current loop sensors with arduino
All the parts used in this tutorial can be found on ncd.io store: